Macro Processor Quick Start Tutorial
Getting Started
- Run HarvEX. Go to the HarvEX Macro Processor page
- In the macro list double-click the preloaded hello_world
macro => The macro script appears in the script edit window.
- Click Execute or use [Ctrl-Return] or [F5] from inside
the script text body => The Script runs, a text appears in the Output
window and a MessageBox is coming up.
First macro : Using the Bid Manager, Storing &
Loading the macro
- Hit the New Button for a fresh macro script.
Type the following lines to the script editor or copy & paste : mark
the stuff > Ctrl-C (=copy) > place cursor to script editor >
Ctrl-V (=paste)
b = bm.GetItem(1234567890) # should be a valid auction ID in bid manager
print b.mybid
- Hit Execute Button => you've extracted a mybid
value from this auction !
- Save this fresh macro to the intern macro list by pressing Save
As => you are asked to enter a macro name; e.g.: my_first_macro
- Store this macro to a disk file : Mouse-right-click on
the macro inside the list and select Save to File from
the popup context menu.
- Loading a macro from disk file : Mouse-right-click somewhere
in the macro list and select Load a Macro from File
Logic Bidding Conditions (Bid Editor)
- A logical sniping condition is the most simply type of
(special) macro: A one-liner function, which can be set in the Bid
Edit Dialog of snipe items in the Bid Manager: If it computes a
zero value, the bid sniping is not executed.
- Test with dummy conditions: In the bid Condition field
in the Bid Edit Dialog enter a "0"; press the "!" button
=> sniper bid would not be executed; enter a "1" (or "---"); press
the "!" button => sniper bid would always be executed.
- Enter a condition in the Condition field by selecting a
template from the drop-down list and edit it. E.g.:
BMItem(123456789).won()
=> the sniper bid on current auction is only exectued when
this other item #123456789 is already won. (Example motive: you want to
win a HIFI amplifier only when the fitting HIFI receiver was already
won)
- Note: the Logic Bidding Condition "Macro" is normally
set in the Bid Edit Dialog for each snipe in the Bid Manager. Yet it
can be also set via
<bid_manager_item_object>.condition = "<condition-macro>" )
from a regular HarvEX script
- Create a new bid sniping in the bid manager
Sandbox Protection
Please Consider: For executing certain critical functions in the
examples below,
you are requested (by an error message) to set the sandbox
restrictions to a lower level.
This "sandbox" level system is useful for protecting yourself from
unintentionally executing critical actions.
|
High Restrictions |
No direct I/O, no critical manipulation in
the HarvEX application. E.g.: changing sniper bid values not allowed |
Medium Restrictions |
Enables non-critical I/O and advanced internal
manipulations. E.g.: manipulating bids, preparing emails in MS Outlooks
draft folder, limited Internet access (ScanItem, ...), reading disk
files, ... |
Low Restrictions |
Enables critical I/O : directly sending out email, free
HTTP/URL access, writing disk files, ... |
Macro Editor
The macro/script editor in HarvEX is Python syntax
aware.
Keyboard Bindings:
- Ctrl-A : mark all
- Ctrl-C/V : copy/paste
- Ctrl-F : rind
- F3 : repeat find
- Ctrl-G : goto line (line number asked from dialog)
- TAB: indent line / marked region
- Shift-TAB : un-indent line / region
- Ctrl-I: create real TAB ( \t )
- RETURN : new-line & indent (aware of Python syntax /
":", open brackets etc. )
- Ctrl-RETURN : execute script
- Shift-RETURN : check script for correct Python language syntax
- F5 : execute script
Macro Language & API
Note: the tag "[HX+]" marks
functions which are only available with HarvEX+ license level - many of
them are yet available during the 14 day trial
period. How to test: You'll get a License Error
message when executing without sufficient license level
The Python Macro Language
HarvEX(+) has a Python Language interpreter built-in. A general
introduction and tutorials for the Python
language itself are found
here.
This chapter shows HarvEX specific Python usage (about: invoking
other macros, returning macro return values, persistent data and more).
The following chapters document the HarvEX Python API.
# '#' starts a comment
CallMacro('hello_world')
# calls another macro (sub macro call)
value = CallMacro('hello_world', a=1, b=2)
# calls another macro with arguments and saves the return value
print a, b # print the arguments which are
# available directly in the namespace of the called macro
raise Return, [1, 'hello world', 2.0]
# exits and returns a list ( to the optional caller of this macro)
exit( [1,'hello world',2.0] )
# alternate return statement
raise Exit # exit a macro (before its natural end)
if MessageBox('really process mails?','Congratulations Mailer',1) != 1:
raise Exit
MessageBox('really process mails?', 'Congratulations Mailer', 1) != 1 or exit()
# the same
if not hasattr(ps,'a'):
ps.a = 0 # ps is the global persistent namespace :
# data persists between consecutive macro calls
ps.a += 1 # increments attribute a in the namespace
print ps.a # prints 1 on first run, 2 on 2nd run, 3 on 3rd run ...
fps = util.FilePersistent( 'C:/data/mypersist.hxp' )
# Defines a disk persistent namespace
# Data persists between HarvEX runs, thus its a Object-Database.
# Only serializable data types should be set in such namespace
if not hasattr(fps,'a'):
fps.a = 0
fps.a += 1 # increments attribute a in the namespace
print fps.a # Prints 1 on first run, 2 on 2nd run, 3 on 3rd run ... iteratively
# even if HarvEX is restarted
time, re, string, sets and math are
pre-imported python modules in HarvEX
scripts. For example use "print time.time();
print math.sqrt(2)"
without importing time or math !
Upon HarvEX startup the macro [init] is called if present.
This init script can be
edited in order to configure a special startup.
Top Level Functions
q = AuctionQuery() [HX+]
Create a query object for usage in the 'SearchItems' Function. See
also GetQueryFromForm.
Example:
q = AuctionQuery()
q.subjects = 'Pentium Computer'
q.completed = True
SearchItems(q)
Query object attributes:
Normal query object attributes can be generated by the "Gen.
Macro" button in the search form.
q.searchurl =
"http://search.ebay.de/search/search.dll?MfcISAPICommand=GetResult&ht=1&query=...."
f
If this attribute is used, this direct search URL is used. All
other attributes are ignored, thus the URL is not computed.
Beep()
Create a 'beep' sound signal.
SearchItems(query/url , adding=0, cached=0,
quiet=0)
[HX+]
Searches Auctions. The output goes to the search page. In case of
quiet=1 the resulting lines are the return value and do not go to the
search page.
Arguments:
query / url : a AuctionQuery() object
or flat url string
adding : 1 = add to existing content in the search page
cached : 0 = no/download; SC_CACHED=cached only; SC_CACHEDORDOWNLOAD
quiet : 0="GUI":same as manual search to search page;
1="return
string": returns only TAB/NEWLINE spaced result string of all
2=same as
0 but no error message boxes;
5="return
list of search item objects": [<OSearchItem>,
..]
Return value: 1 = search result OK (when quiet=0/2); or list of
<OSearchItem>
objects (when quiet=5)
Example:
lresult = SearchItems(q, quiet=5)
q = GetQueryFromForm() [HX+]
Create a query object (see AuctionQuery) pre-filled from the
current selections in the Search Form page ! Use full
for creating "Multi-Searcher" macros
Arguments:
[q] : query object
Example:
q = GetQueryFromForm()
print q # see attributes you may alter in q !
rc = MessageBox( text="test",
title="Macro Message", opts=0)
Displays a MessageBox and waits for user confirmation. This
function may not be used in background tasks
(Threads). Use AMessageBox in order to not
block the programm flow.
Arguments:
opts
: 0=OK Button, 1=OK+CANCEL Buttons, 3=YES+NO+CANCEL Buttons, 4=YES+NO
Buttons, 2=ABORT+RETRY+IGNORE Buttons, 16=Error Box, 48=Exclamation
Box, 64=Information Box (options can be combined with the "|" (OR)
operator)
[return value] : 1 = OK
pressed, 2 = CANCEL pressed, 3=ABORT pressed, 4=RETRY pressed, 5=IGNORE
pressed, 6 = YES pressed, 7 = NO pressed
Example:
if 1 != MessageBox("This is a message", "Hello World", 1 | 48):
print "user canceled the operation"
raise Exit
print "lets continue ..."
util.AMessageBox(text, title="Message",
opts=64)
Raises assynchronously a Message/MessageBox in the GUI (HarvEX
multi-message display system). Doesn't block program flow or wait for
user confirmation. No return Value. This function can be used in scheduled jobs and background tasks (Threads) too.
Arguments:
opts : see MessageBox
Example:
util.AMessageBox("a small problem occurred, but we don't stop", "Warning", 48)
print "lets continue ..."
oscan = ScanItem( itemno, cached=SC_CACHED )
Arguments:
cached : SC_CACHED, SC_DOWNLOAD [HX+],
SC_CACHEDORDOWNLOAD [HX+]
[oscan] : <scan item object> with attributes :
item,title,endtime,currentbid,... like Scan Page / Config table
columns.
See also:
<oscan>
object
Example:
o = ScanItem(1234567890)
print o.nbids
Top Level Objects
bidmanagerpage
The Bid Manager Object
Shortname: bm
Access / Examples:
bid = bm.GetItem("1234567890") # returns a <Bid> object
print bid.get_mybid(),bid.title
bid = bm.AddItem("1234567890") # creates new or returns existing
bm.DelItem("1234567890")
items = bm.GetItems() # returns item numbers of all Bid Manager items
items = bm.GetSelectedItems()
for bid in bm.Iter():
print bm.title #iterates directly over bid objects
bid = bm.GetItem("1234567890")
bid.mybid = 7.03
bid.set_snipe()
bid.ExecBid(no_msg=True)
See also:
<bid>
object
Bid Manager Functions
AddItem
scanpage
Shortname: scp
Examples:
scanpage.Show() #shows the scan page
scanpage.SetMatrix( [ ['abc','def'],['ghi','jkl'], ... ] ) [HX+]
scanpage.SetLines( [ 'abc\tdef' , 'ghi\tjkl',... ] ) [HX+]
m = scanpage.GetMatrix()
print m[0,0]
See also:
<oscan>
object
searchpage
Shortname: sep
Examples:
searchpage.Show() #shows the search page
searchpage.SetMatrix( [ ['abc','def'],
['ghi','jkl'], ] ) [HX+]
macropage
Shortname: mp
Examples:
macropage.Show() #shows the macr page
macropage.ClearOutput()
persistent
carries variable values over the execution time of a macro.
Shortname: ps
Example:
try: persistent.my_globalcounter
except: persistent.my_global_counter = 0
persistent.my_global_counter += 1
print persistent.my_global_counter
Modules
util
Utility module functions.
ls = util.ScanOutlook( folder,
filter=..., pattern=..., errorlevel=1)
[ HX+ required for scanning
more than 10
emails ]
Arguments:
folder : MS Outlook folder in directory slash (/) notation
like "inbox/somewhere/ebay_in". "inbox" is language independent
lockation for the Mail Inbox, the rest of the dirs are identical with
your Outlook folder names
[ls] : list of strings of result lines; strings tab spaced
columns according to the pattern.
Example: ( see more real world example in the template
section ! )
# retrieve buyer email addressses from german ebay.de emails
outlookfolder = "inbox/ebaynew"
subjectcontains = "Sold Item|Verkaufter Artikel" # read only emails containing this
columns = '$item;$email;$ebayname;$fullname;$street'
pa_all = [
'(\d{8,10})', # extractor pattern for: item no.
'Käufer:.*?([\w.+-]+@[\w.+-]+)', # email address
'Mitgliedsname:(.*)',
'Name:(.*)',
'Straße:(.*)',
]
l = ScanOutlook(
folder=outlookfolder,
filter=subjectcontains,
pattern=pa_all,
errorlevel=0
)
print l
scanpage.SetList( l, headers=columns )
scanpage.Show()
f = util.Str2Money( s )
Example:
print util.Str2Money('2,345.50')
print util.Str2Money('2.345,50')
s = util.Money2Str( floatvalue )
s = util.Money2StrUS( floatvalue )
s = util.Money2StrDE( floatvalue )
( The first one uses the current country settings. )
Example:
print util.Money2Str(2345.50')
print util.Money2StrDE(2345.50')
s = util.ExtractCurrency( "EUR 230,00" )
s = util.ExtractMoney( "EUR 230,00" )
=> "EUR"
=> "230.00"
works for any currency and country specific format ( , . style
etc. )
s = util.matrix2text( matrix )
Parameter:
matrix : list of lists like
[[11,12],[21,22],[31,32]]
Example:
util.matrix2text([[11,12],[21,22],[31,32]]) #=> Tab & Newline spaced text
m = util.lines2matrix( lines )
Turn tab spaced lines into a list-of-list-matrix.
Parameter:
matrix : list of lists like [[11,12],[21,22],[31,32]]
Example:
util.lines2matrix(['abc\tdef','ghi\tjkl') #=> [['abc','def'],[...]]
util.isfile(path)
util.isdir(path)
util.remove(path)
os functions within sandbox.
TL = util.ReadTurboListerCSV( fname ) [HX+]
Read and understand a TurboListerCSV (template) file. Details:
See the extensive example above.
It is not wise/possible to create a TurboLister file from scratch,
because there are around 100 fields to be set. The field layout
may also change in future releases of TurboLister.
Best practice: Use a template file already exported from TurboLister (TL/menu/File/Export
to CSV) and load it with util.ReadTurboListerCSV, then manipulate
the fields in this template and write the final TL CSV file and
re-import in TurboLister.
Using a TL Object:
TL = util.ReadTurboListerCSV( "C:/data/mylistings.csv" )
print TL.headers # prints the names of available Listing attributes
t = TL.copy(0) # take the first listing ( #0 )
print t['Title'] # => 'Nice Auction'
t['Description'] = 'my nice auction: this is my new text'
t['Category 1'] = '1234' # set the new category number
TL.clear() # clear all other listings,
TL.add(t) # set only the modified and ..
TL.write("C:/data/modified_listings.csv")
# .. create file for re-import in TurboLister
SetMenuMacro( title, macroname_or_function, id=None , pos=1000 )
Set a menu item in the "+Tools" menu of the HarvEX GUI. 'id =
None' always appends. 'id = 123' writes or overwrites a certain item.
using 'pos' you can control the exact position of the menu item.
util.SetMenuMacro('&Filter Search Results with 1..4 bids on [filter_search]',
'filter_search',
202)
def hello():
print "hello"
print "this is a function"
util.SetMenuMacro( '&Hello', hello )
util.SetMenuMacro( '&Hello', lambda:MessageBox("Hello HarvEX User") )
mailitem = CreateOutlookMail( subject="hello", text="this is a
message",
toaddr="hello@hello.world.net" , send=0 )
[HX+]
Set a menu entry in the "+Tools" menu of the HarvEX GUI.
folder =
util.GetOutlookFolder(folder='inbox/my/sub/folder') [HX+]
Return a folder object from folder path.
url_data = util.GetItemPicture(
item, url=1, index=0): [HX+]
Returns item picture data; or None if not existing.
url: 1=return url only; 0=return raw data, 2=return
(image_content_type, raw_data)
index: 0=standard preview image; 1=tumbnail icon; 2=full-image 1st;
3=full-image 2nd; ..; -1=last full-image; -2=second to last full-image
item = bm.GetSelectedItems()[0] # '123456789012'
print "GetItemPicture", item
for ix in range(10):
url = util.GetItemPicture(item, index=ix)
print ix, ":", url
if not url:
break
imgtype, data = util.GetItemPicture(item, url=2, index=1) # thumbnail icon
print imgtype, len(data)
open('C:/tmp/thumb-' + item + '.jpg', 'wb').write(data)
w = util.WaitCursor():
[HX+]
A WaitCursor will be displayed until the w object disapears.
s = util.GetUrl("http://xx.yy.com/mydoc.html") [HX+]
Gets the content of a url page
s = util.GetUrlEbaySignin(ebayurl [,userpwd='otheruser']) [HX+]
Gets the content of a url page at eBay where eBay Sign-In is
required. the main ebay account from config page or 'otheruser' is
used. These passwords have to be registered in menu/Config/Mulitple
Accounts.
s = util.GetClipboardText()
=> "Hello, this is a Text from Clipboard"
util.SetClipboardText("Hello!")
Sets the Windows clipboard text
s = util.GetMainEbayUser()
=> "my_nice_account"
t = util.GetSyncedTime( bmitem.endtime )
Translates a linear server (auction) time to the local
synchronized time ready for comparing it to time.time()
(current PC time) values.
s = util.GetSimpleInput(name="", s="")
Requests an input string from the user. name is the
question/term presented.
name : promt string
s : pre-filled string
util.SetAuctionRetrieveHandler( handler_func,
id=1) [HX+]
Installs a function to be called after auction data update in
bid manager. The handler_func is called with one parameter bid
: a bid manager
bid
object. For example: Such handler may be used to post-process
auction data after retrieving from ebay. E.g.: generate computed
comments;
Several handlers maybe installed with different id's - handlers
with lowest id's are called first. Setting a handler again
with the same id overwrites. Setting None or lambda
bid:None uninstalls the handler with ID id.
util.Schedule( macro_or_func,
period_or_pattern=2, id=None ) [HX+]
Installs a periodically executed background macro or function.
It is recommend to use a function, because of 2 reasons: 1) A function
has far less time overhead when being called. 2) You can use the
persistent namespace of your macro.
macro_or_func : function or macro name or None
(None = kill the job for a certain id)
period_or_pattern : Period in seconds
id : e.g. id=123 : Scheduling a new job (or None)
with same id replaces the old job with that id
Example "Command execution through file interface & polling":
#init 777
cmd_in = "hx-cmd-in.txt"
cmd_out = "hx-cmd-out.txt"
def poll():
if util.isfile(cmd_in):
cmd = open(cmd_in).read()
util.remove(cmd_in)
ret = eval(cmd)
open(cmd_out, 'wb').write(repr(ret))
util.Schedule(poll, 1.0, id=177)
# schedules the function poll to be called every second
bkcall =
util.BackgroundCall((self, func, args=(), kwargs=None) [HX+]
( For expert use only! Most time you are better off with util.Schedule )
Runs the function func in background - as extra thread / task /
job / service. The task may run as long as HarvEX is running.
Communication to the func running in background can be done via
any variables or parameters accessibly by func.
If func is intended to run as sort of long time service (and
not
just compute a result as fast as possible), it should use
time.sleep(..) in order to not "loop" at maximum CPU load.
args (tuple) and/or kwargs (dict or None) are
parameters passed to func.
[return value]: A object representing the background
call execution:
<bkcall> Object members:
bkcall.is_done() : returns 1 if func
finished regularly, 2 if func terminated by exception, 0 if func
didn't finish so far.
bkcall.get_return(timeout=10.0) : returns the return value
of the executed function func. Raises an exception if func
terminated by exception.
Example:
# starts a background task
if hasattr(ps, 'mybkcall') and not ps.mybkcall.is_done():
print "task already/still running! I'm requesting a stop"
ps.mybkcall_run = False # request a stop
exit(-1)
def background_task(a, b, c):
print a, b, c
while ps.mybkcall_run:
print "background_task"
util.AMessageBox("I don't waste CPU time, but raise a message every 10 seconds",
"background_task")
time.sleep(10.0) # do not consume CPU time while doing nothing
return a + b + c
ps.mybkcall_run = True
ps.mybkcall = util.BackgroundCall(background_task, (1,2), dict(c=3))
print "started."
# request termination of background_task and tell return value
ps.mybkcall_run = False # request stop
r = ps.mybkcall.get_return(timeout=3.0)
print "the background task return value was:", r
util.COMDispatch( com_name
) [HX+]
Retrieve a COM Interface in order to control other applications
like MS Excel, MS Word, etc. on this computer.
xl = util.COMDispatch("Excel.Application")
xl.Visible = 1 # want to see it live!
workbook = xl.Workbooks.Add() # new .XLS workbook
sheet = workbook.Sheets(1) # get first Sheet of that
sheet.Cells(1,1).Value = "First Cell!"
sheet.Cells(2,1).Value = 1.0 # 2nd row, 1st column
sheet.Cells(3,3).Value = "=SQRT(2+3)" # Excel computed expression
tab=[
[1, 2, 3, 4],
[5, 6, 7, 8],
]
sheet.Range(sheet.Cells(4,1), sheet.Cells(5,4)).Value = tab
# write some Bid Manager values out to Excel :
for ix, item in enumerate(bm.GetSelectedItems()):
bid=bm.GetItem(item)
sheet.Cells( 7+ix, 1 ).Value = bid.item
sheet.Cells( 7+ix, 2 ).Value = bid.title
sheet.Cells( 7+ix, 3 ).Value = bid.mybid
workbook.Save()
Objects
Bidmanager Item Object <bid>
Get a bid object like:
bid = bm.GetItem("1234567890")
for bid in bm.Iter():
print bid.title
Setting of certain attributes requires [HX+]
<bid> Object Attributes:
item = '' # item id e.g. '123456789012' [read-only]
title = '' # item title [read-only]
endtime = 0 # item end time e.g. 1324216383.0 [time.time()] [read-only]
comment = '' # comment string [HX+]
mybid = 0.0 # my maximum bid e.g. 50.11 [HX+]
quant = 1 # number of items to bid on in this auction [HX+]
state = 1 # item state [integer value 0..9] [HX+]
# INACTIVE,INACTIVE2,SNIPE,BIDOK,OUTBID,TOOLATE,FAILURE,MISSED,WON,GBLOCK
currentbid = 0.0 # current bid/price e.g. 47.5 [read-only]
currency = '' # e.g. '$' ; 'EUR' [read-only]
binprice = 0.0 # buy now price if available e.g. 60.0 [read-only]
nbid = '--' # current number of bids on this item e.g. '3' [read-only]
ship = '' # shipping price value/info e.g. '5.90'
seller = '' # seller id e.g. "Sir.Prise" [read-only]
high_bidder # current high bidder id (mangled) [read-only]
finished = False # non-zero if the auction/item has ended [HX+]
group = '' # bid group e.g. "bike" ; "bike(2)" [HX+]
on_sale = 1 # number of items on sale e.g '1' ; '-' [read-only]
alarmdt = 300 # alarm seconds before auction end (0=OFF)
userpwd_ex = None # tuple or None e.g.: ("ebay_freak","secret_passwd") [HX+]
condition = '' # advanced bidding condition formula / script [HX+]
# e.g. 'not BMItem(123456789012).won()'
ibidtimemargin = 0 # item bid time marging [seconds] (0 = use global default)
won() # returns True if item was won
set_snipe(standalone=True, se=False) [HX+]
# shortcut for setting sniping ON/OF and/or SE-snipping ON/OFF
ExecBid(no_msg=False, bidcmd=0) [HX+]
# Execute a Bid/auto-Buy-Now (bidcmd=0) or force Buy-Now (bidcmd=1)
# example: bid.ExecBid(no_msg=True)
GetShippingFloat() # returns shipping costs as float, 0.0 if unknown
Update() # updates data from server/market
Scan Item Object <oscan>
Get a scan
item object like: oscan=ScanItem("1234567890",SC_CACHEDORDOWNLOAD)
<oscan> Object Attributes & Default Values:
item = '' # item id e.g.: '123456789012'
title = "?" # item title e.g.: u'Wonderful eBay Item'
words = "" # matched special terms
titlewords = "" # = title + words
finished = False # non-zero if the auction/item has ended
on_sale = 1 # Quantity (how many items are on sale)
privat = False # if its a privat auction
bid = '0.0' # current bid/price e.g. '40.55'
bidq = '?0.0' # e.g. '40.55' ; '?40.55' (? = uncertain/not finished)
buynow = False # if its a buynow item
binprice = 0.0 # buy now price, if available
currency = '?' # e.g.: 'EUR' ; '$'
currencybid = '??' # e.g.: 'EUR 40.55'
nbid = 0 # number of bids up to now
sold = '' # number of sold items if nonzero
available = '' # number of available items if nonzero
da = '' # e.g. '18-Dec-2011'
ti = '' # e.g. '13:53:03'
dati = '' # e.g. '18-Dec-2011 13:53:03'
dati_local = '' # '18.12.11 14:53:03' (local timezone)
timezone = '' # EST ..
own = False # if we probably won/bought it [unreliable]
endtimetime = 0 # auction end time e.g. 1324216383.0 (->time.time())
scantimetime = 0 # last HarvEx scan time e.g. 1324219247.021
high_bidder = "" # (mangled) id of current highbidder e.g. 'e***x'
seller = "?" # seller id e.g. 'Sir.Prise'
seller_rating = '' # e.g. '100%'
sellerhighbidder = "" # 'Sir.Prise -> e***x'
ship = "???" # shipping costs e.g.: '6.50'
total = "?" # current total price bid+ship e.g. '47.05'
html_path = '' # path of cached html on disk
charset = 'utf-8' # charset of HTML
GetBidFloat() # returns current bid/price as float or raises ValueError
GetShippingFloat() # returns shipping costs as float or raises ValueError
html : property # [str/unicode] complete item page html
text : property # [str] description part of item page html
textW : property # [unicode] description part of item page html
Search Item Object
<OSearchItem>
Get a search item object like:
l_osi = SearchItems(q, quiet=5)
osi = l_osi[4]
print osi.title, osi.bid, osi.shipping
<OSearchItem> Object Attributes & Default Values:
item = '' # item id e.g.: '123456789012'
bid = '' # current bid/price e.g. '40.55'
buynowprice = '' # buy now price, if available
buynow = False # if its a Buy Now item
currency = '?' # e.g. 'EUR' ; 'US$'
nbid = '-' # number of bids up to now
shippingprice = property(lambda self:self.shipping) # shipping price #$pycheck_no='classic'
shipping = '' # shipping price
remtime = '' # time remaining for this auction/item
htmlitem = '' # HTML code of this search item
paypal = '' # __nonzero__ if paypal payable item
# [if Paypal option in MyEbay search result customizations AND LogonEbaySearchSite done]
seller = '' # Seller User ID
# [if Seller User ID option in MyEbay search result customizations AND LogonEbaySearchSite done]
get_nbid() # returns integer of nbid or 0, if none
get_bid() # returns float current bid/price value
GetBidFloat() # (alt. name)
get_buynowprice() # returns float buy now price or 0.0 if none
GetBuyNowPrice() # (alt. name)
get_shippingfloat() # returns float shipping costs value, 0.0 if 'Free'; raises ValueError if unknown
GetShippingFloat() # (alt. name)
Auction Query Object <query>
Note The "Generate Query Template" button in the HarvEX
Search
Form pane helps to generate a query template.
<query> Object Usage Example:
query = AuctionQuery()
query = GetQueryFromForm() # fetch from HarvEX Search From
query.cat = "#1234" # Category Id 1234
SearchItems(query)
q = AuctionQuery()
q.searchurl = "http://search.ebay.com/..." # search from a URL
q.maxpages = 10
for osi in SearchItems(q, quiet=5):
# iterate over result list of <OSearchItem>'s
print osi.title, osi.get_nbid()
<query> Object Attributes & Default Values:
subjects = "" # search term(s) e.g. "dvd player"
excl = "" # words to exclude e.g. "refurbished used"
cat = '' # category ID e.g. '1234' ; '#10614 Audio & Hi-Fi'
region = "0" # region ID ; "0" = all
minPrice = 0.0 # minimum item price
maxPrice = 0.0 # maximum item price
anddesc = False # True = search item title and description
searchopt = '1' # '1'=AND (all words) 2=OR (any of the words)
# '3'=exact match/sentence '4'=exact words
completed = False # True = run a completed items search
daysback = 30 # completed search: max. number of days back
# (still not supported well by eBay (1/2012))
auctions = False # True = search Auction items (only)
buynow = False # True = search Buy Now items (only)
morethan1 = False # True = find multi-items/auctions only
multiple_min = None # min and .. e.g. 2
multiple_max = None # max multi items e.g. 5
paypal = False # find only PayPal payable items
inclstores = False # search in stores as well
sortby = 0 # sort order index [0..10]
resultsperpage = "50" # max number of items per server result page
worldlocation = 0 # 0=preffered; 1=located_in; 2=available_to
available_to = "0" # country ID
located_in = "0" # country ID
distance = '' # kilometers / miles e.g. '20'
sellers = '' # include/exclude sellers e.g. 'seller1, seller2, Sir.Prise'
sellers_exclude = False
# if True, then `sellers` means: exclude
searchurl = '' # ! overrides the search URL at all. If set, then
# none of the above query attributes take effect
maxpages = 1 # max number of result pages to retrieve
New API suggestions:
feedback@xellsoft.com.
Continue reading the
FAQ, Tips &
Trick
page.
Power Macros
This chapter describes the Standard Power Macros
for HarvEX / HarvEX+. They add
powerful bulk
processing options and/or other new features to HarvEX
Power Macros with extra Homepage
eBay Auto Search & Snipe/Bid
:: Power Macro
eBay Auction Search Collector ::
Power Macro
Excel Bulk Sniping :: Power Macro
Sets up sniping jobs in the bid manager using bulk data from an
Excel Table
or CSV/TabSV file.
Columns: itemno, my_max_bid, [group, group-N, margintime, SE_flag
Price : US$ 30
Use Case : Bulk sniping after preparing jobs in Excel/table
files
Status : v1.05 ; Existing / Widely Used; ships with 1 business
day.
Buy :
Contact:
sales@xellsoft.com
Screenshot Pieces
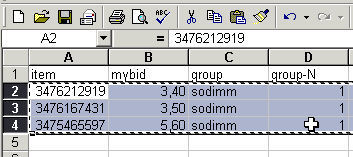
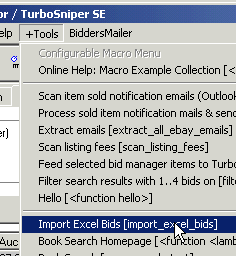
Details / Usage:
- Set up an arbitrary amount of bid tasks in an Excel table.
Table columns are: <item number>, <my maximum bid> and
optional: <bid-group> + <group-N> (number of items you want
to win in this group)
- You copy the Excel lines to clipboard (press Ctrl-C in Excel)
- Hit Menu/+Tools/Import Excel Bids : Sniping bids are imported
- Works internationally with any float number format in the
mybid columns
Scan Item Specifics :: Power Macro
This Power Macro extracts extra standardized "Item Specifics" of
auctions/items - as they are shown on top of the Description section of
eBay
Item Pages. The existence of Item Specifics depends on the eBay
category of the item. The new
attributes can be rendered as new columns in the Scan pane results
table, and are available for other macros as new <OScan>
attributes.
In addition, extra item information outside of the standard
Item Specifics can be extracted too as shown
in the Macro Head section below.
Price : US$ 20
Status : v2.0 ; Existing / In use; Ships within 1 business
day.
Buy :
Contact:
sales@xellsoft.com
Macro Head / Configuration:
#!init 777 # auto-run this macro at HarvEX boot time
# HarvEX+ Power Macro [scan_itemspecifics] v2.0:
#
# Installs a scanner for additional Item Specifics or other attributes from an auction/item page.
# Exposes them as new <OScan> object attributes plus adds a combo-attribute 'Itemspecifics'
# To add them in HarvEX GUI Scan results pane, add the new attributes in Menu/Config/TableColumnFormats like:
# "$item;...;$Itemspecifics;$Size"
# In other macros you can use the new attributes for example after ScanItem(...) like:
# mysize = oscan.Size
# MACRO CONFIGURATION
# Variables from the Item Specifics section, which shall be set as extra <OScan> (Scan Column) attributes
extra_scan_vars = [
'Condition',
'Brand',
'Color',
'Size',
]
# extra things to scan outside of the Item Specifics section
extra_scan_patterns = [
( 'Location', r'(Item location):.*?>([^<]+)<' ),
]
...
Screenshot:
eBay Smart Bulk Feedback Tool
(Positive-on-Positive Feedback) :: Power Macro
Smart bulk feedback tool for HarvEX / HarvEX+. Supports bulk-putting
of eBay feedback - particularly positive upon positive feedback
selectively. Yields warnings for negative/neutral Feedback.
Price : US$ 20
Use Case : Posting
feedback on eBay after bulk purchases effectively - positive feedback
for positive feedback selectively.
Status : Existing / In use. Ships within 1 business day.
Buy :
Contact:
sales@xellsoft.com
Details / Usage:
- Load macro with Menu/File/LoadMacro
- Execute the macro once for installing the new Menu Command. (will
be done automatically after HarvEX start from now on)
- Run Menu/Tools/LeavePositive2PositiveFeedback(Bulk Operation) etc.
- Follow the exection flow in the Macro Magic Web Browser ...
Bulk Extract Auction Details ::
Custom Power
Macro
Description: Searching and bulk extraction of particular details of
auction/item descriptions to a table / output file.
Details can be standard category details withing certain eBay folders
(e.g. book
attributes, ISBN number ...), or can be things found by
expression
matching etc.
Typical Price : US$ 30;
depends
on specific task.
Example Use Cases : Bulk extract sports ticket data for further
processing
in Excel; re-search book prices in completed auctions;
Status : Existing template, will be
adapted to the
need of the user. Ships within 1 .. 5 business days.
Buy / Question :
sales@xellsoft.com
Bulk Feed Data to TurboLister ::
Custom Power
Macro
Description: Grabs product
data from a Reseller
Web Page, XML, CSV ... and pre-computes eBay listings for TurboLister
Typical Price : US$ 100 ; depends on specific task.
Use case : eBay re-selling
Buy / Question :
sales@xellsoft.com
Details:
- Scans a certain list of products and prices from this reseller.
- A custom algorithm detects changed prices and interesting products
- Feeds TurboLister with pre-filled listing & computed
sell prices for selected products from this reseller;
- After a short check & edit these items can be listed on eBay
using TurboLister with a very small amount of work !
Ask for other custom solutions:
Request
Form
Macro FAQ
" What can I do and not do with macros "
- HarvEX provides a full sized object oriented scripting system,
but it is very easy to use for smaller tasks.
You can program and automate nearly all tasks of interest for eBay bidders
and seller. You can automate and manipulate most aspects of HarvEX
through the API. Table computation & manipulation is especially
easy. You can do free web programming ( including automated reading,
parsing and sending of emails, calling and parsing HTTP/HTML from eBay
or from any URL, ... ), writing & reading tables and files, etc.
You can do user interface programming. You can program background
processes & scheduled tasks. A macro connected browser is built
into HarvEX. The API is continuously growing.
- You can also program tasks, which have not much to do directly
with auctions / eBay - its more easy than with most other programming
systems - The HarvEX macro language is especially strong at internet
programming, manipulating files, tables and automating email, MS
Outlook, Excel, Databases.
Its often more efficient & easy to use HarvEX macros in comparison
to using a low level software developmet kit: A stable application
framework, functional table grids, a display system, a task system and
a error handling system are already in place.
Thus this tool offers a very fast edit-run cycle.
" What language syntax is used for HarvEX macros? "
- It is the
Python
language: A modern
state-of-the-art object orient script language, which encourages
readability and short code.
- For beginners
getting started
is more easy than
even BASIC.
- For power users this language offers more flexibilty and
syntactic power than languages like Javascript, PHP, Perl & C#
" How do I quickly learn this macro system? "
- Trial & Error
(The HarvEX macro "sandbox" is very robust and points you quickly to
the problems.)
- Go through the
HarvEX
Macro Tutorial and the
Python ultra short tutorial
- Walk through the most interesting examples above.
- Alter the code, execute & see what happens
- Have a walk through the HarvEX API description